Some tips on splicing and dicing Pandas DataFrames¶
[1]:
import datetime
print('Last updated: {}'.format(datetime.date.today().strftime('%d %B, %Y')))
Last updated: 05 July, 2019
Outline:¶
Pandas DataFrames (two-dimensional) and Series (one-dimensional) data structures are extensively used in the brightwind library for the storage, transfer and display of data. A simple explaination is a Series is simply a single column of data which can have a index and a DataFrame is multiple columns of data with an index. Both are explained in more detail here:
https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.html
For an easier intro to them you could check out the below link. Needless to say, Pandas makes Python a powerful data analysis tool.
https://www.geeksforgeeks.org/python-pandas-dataframe/
This tutorial will give a basic introduction to using these data structures and outline how to:
Seperate specific columns from DataFrames into new DataFrames and Series
Select specific ranges from DataFrames and Series using the index
Search for a specific entry in a DataFrame or Series
1: Selecting Columns¶
Data can be read into DataFrames easily from excel and csv files easily using the brightwind functions load_csv()
and load_excel()
. In the example below, data is read from the csv file demo_data into the DataFrame data.
[2]:
import brightwind as bw
data = bw.load_csv(r'C:\Users\Stephen\Documents\Analysis\demo_data.csv')
data.head(5)
[2]:
Spd80mN | Spd80mS | Spd60mN | Spd60mS | Spd40mN | Spd40mS | Spd80mNStd | Spd80mSStd | Spd60mNStd | Spd60mSStd | ... | Dir78mSStd | Dir58mS | Dir58mSStd | Dir38mS | Dir38mSStd | T2m | RH2m | P2m | PrcpTot | BattMin | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | |||||||||||||||||||||
2016-01-09 15:30:00 | 8.370 | 7.911 | 8.160 | 7.849 | 7.857 | 7.626 | 1.240 | 1.075 | 1.060 | 0.947 | ... | 6.100 | 110.1 | 6.009 | 112.2 | 5.724 | 0.711 | 100.0 | 935.0 | 0.0 | 12.94 |
2016-01-09 15:40:00 | 8.250 | 7.961 | 8.100 | 7.884 | 7.952 | 7.840 | 0.897 | 0.875 | 0.900 | 0.855 | ... | 5.114 | 110.9 | 4.702 | 109.8 | 5.628 | 0.630 | 100.0 | 935.0 | 0.0 | 12.95 |
2016-01-09 17:00:00 | 7.652 | 7.545 | 7.671 | 7.551 | 7.531 | 7.457 | 0.756 | 0.703 | 0.797 | 0.749 | ... | 4.172 | 113.1 | 3.447 | 111.8 | 4.016 | 1.126 | 100.0 | 934.0 | 0.0 | 12.75 |
2016-01-09 17:10:00 | 7.382 | 7.325 | 6.818 | 6.689 | 6.252 | 6.174 | 0.844 | 0.810 | 0.897 | 0.875 | ... | 4.680 | 118.8 | 5.107 | 115.6 | 5.189 | 0.954 | 100.0 | 934.0 | 0.0 | 12.71 |
2016-01-09 17:20:00 | 7.977 | 7.791 | 8.110 | 7.915 | 8.140 | 7.974 | 0.556 | 0.528 | 0.562 | 0.524 | ... | 3.123 | 115.9 | 2.960 | 113.6 | 3.540 | 0.863 | 100.0 | 934.0 | 0.0 | 12.69 |
5 rows × 29 columns
Once this data is loaded, the different columns and rows in the dataframe can be isolated for use in other calculations. To isolate the first column, Spd80mN, from the DataFrame data into the Series Wspd80mN the command is:
[3]:
Wspd80mN = data['Spd80mN']
The series Wspd80mN can then be easily passed into a function such as monthly_means()
:
[4]:
bw.monthly_means(Wspd80mN)
[4]:
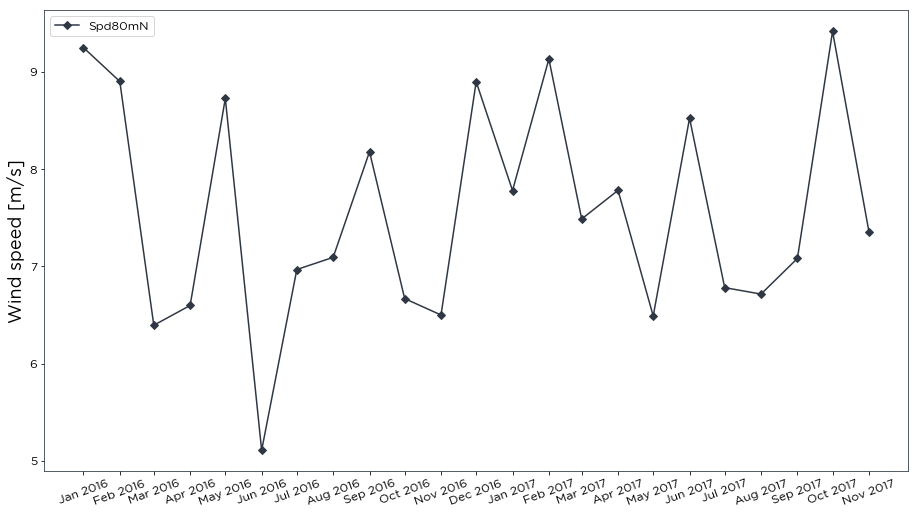
Similarly, you can select a few columns at the same time.
[5]:
bw.monthly_means(data[['T2m', 'BattMin']])
[5]:
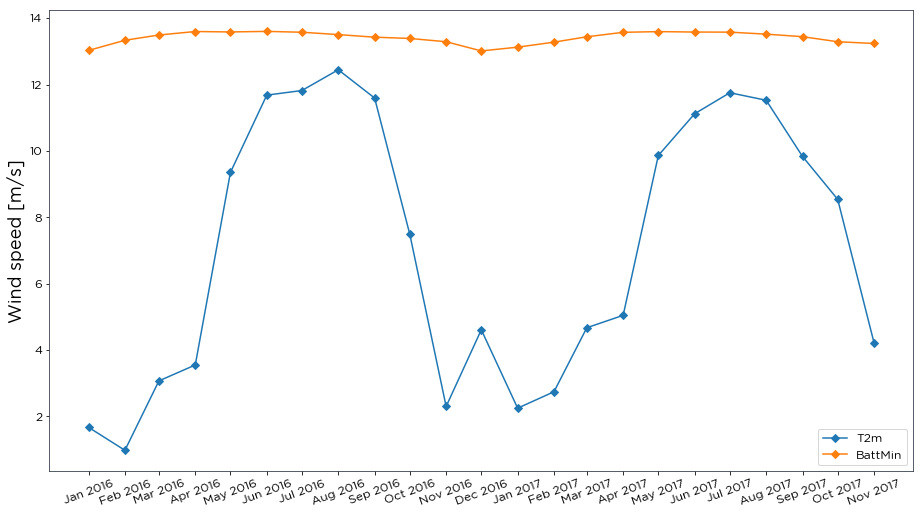
Another way to select a single column is to use the data.Spd80mN
. This only works if you column names have no spaces in them.
[6]:
bw.monthly_means(data.Spd80mN)
[6]:
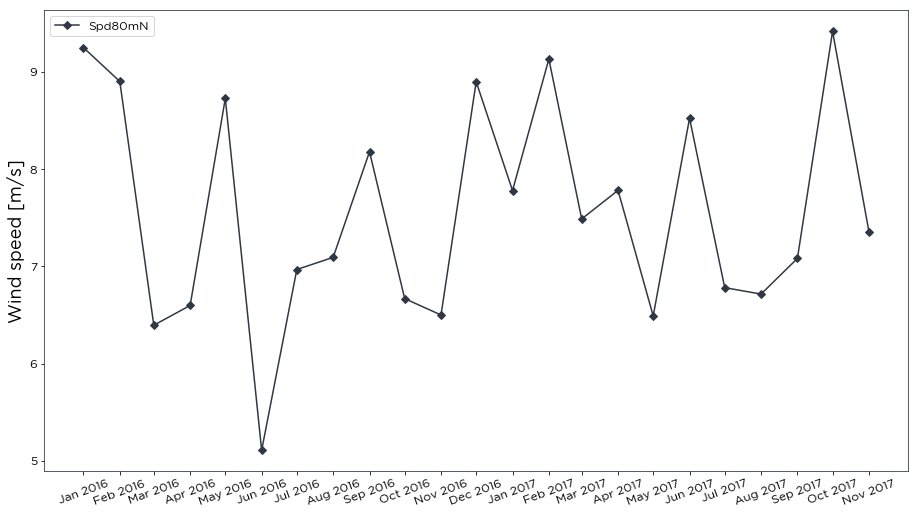
Step 2: Selecting Ranges¶
Ranges, or rows, from within a Series or DataFrame can be seleced. The brightwind library always asigns the index of the DataFrame to be a datetime data type making it much easier to work with the DataFrame for wind analysis purposes. For example, to select data points between two dates for the data DataFrame we loaded in:
[7]:
data['2017-01-01':'2017-02-01']
[7]:
Spd80mN | Spd80mS | Spd60mN | Spd60mS | Spd40mN | Spd40mS | Spd80mNStd | Spd80mSStd | Spd60mNStd | Spd60mSStd | ... | Dir78mSStd | Dir58mS | Dir58mSStd | Dir38mS | Dir38mSStd | T2m | RH2m | P2m | PrcpTot | BattMin | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | |||||||||||||||||||||
2017-01-01 00:00:00 | 5.876 | 5.858 | 5.747 | 5.744 | 5.605 | 5.558 | 1.160 | 1.109 | 1.186 | 1.158 | ... | 7.025 | 275.2 | 0.0 | 324.900 | 6.451 | 3.077 | 99.7 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:10:00 | 5.911 | 5.898 | 5.539 | 5.621 | 5.324 | 5.345 | 1.114 | 1.079 | 1.005 | 0.977 | ... | 7.498 | 275.2 | 0.0 | 319.600 | 9.160 | 2.915 | 99.8 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:20:00 | 7.004 | 6.967 | 6.741 | 6.797 | 6.645 | 6.634 | 1.038 | 0.998 | 1.042 | 1.033 | ... | 6.283 | 275.2 | 0.0 | 316.500 | 7.228 | 2.865 | 99.8 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:30:00 | 7.079 | 7.067 | 6.832 | 6.881 | 6.792 | 6.801 | 0.705 | 0.667 | 0.707 | 0.665 | ... | 4.661 | 275.2 | 0.0 | 319.100 | 5.279 | 2.693 | 97.8 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:40:00 | 6.796 | 6.765 | 6.684 | 6.742 | 6.658 | 6.685 | 0.794 | 0.785 | 0.812 | 0.792 | ... | 5.036 | 275.2 | 0.0 | 317.300 | 6.070 | 2.471 | 98.4 | 967.0 | 0.0 | 12.71 |
2017-01-01 00:50:00 | 8.380 | 8.350 | 8.070 | 8.170 | 7.861 | 7.915 | 0.999 | 0.949 | 0.920 | 0.871 | ... | 5.734 | 275.2 | 0.0 | 314.400 | 5.881 | 2.329 | 99.1 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:00:00 | 8.910 | 8.870 | 8.310 | 8.400 | 7.992 | 7.991 | 0.751 | 0.717 | 0.809 | 0.775 | ... | 3.719 | 275.2 | 0.0 | 315.900 | 4.887 | 2.137 | 98.5 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:10:00 | 8.490 | 8.460 | 8.010 | 8.050 | 7.507 | 7.526 | 0.720 | 0.665 | 0.779 | 0.756 | ... | 4.544 | 275.2 | 0.0 | 323.500 | 5.816 | 2.006 | 99.0 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:20:00 | 7.116 | 7.096 | 6.755 | 6.739 | 6.410 | 6.354 | 0.719 | 0.678 | 0.741 | 0.700 | ... | 5.676 | 275.2 | 0.0 | 326.100 | 5.782 | 1.783 | 98.9 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:30:00 | 8.060 | 8.070 | 7.415 | 7.446 | 6.812 | 6.814 | 0.867 | 0.835 | 0.912 | 0.827 | ... | 5.352 | 275.2 | 0.0 | 323.600 | 6.129 | 1.742 | 99.6 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:40:00 | 9.150 | 9.170 | 8.480 | 8.300 | 7.883 | 7.721 | 0.738 | 0.685 | 0.789 | 0.750 | ... | 5.377 | 275.2 | 0.0 | 330.600 | 6.721 | 1.763 | 99.8 | 966.0 | 0.1 | 12.70 |
2017-01-01 01:50:00 | 7.837 | 7.820 | 7.132 | 6.473 | 6.608 | 6.100 | 0.837 | 0.834 | 1.013 | 1.140 | ... | 14.100 | 275.2 | 0.0 | 339.700 | 6.033 | 1.783 | 99.3 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:00:00 | 6.234 | 6.149 | 5.446 | 4.847 | 4.863 | 4.624 | 1.133 | 1.067 | 0.962 | 0.795 | ... | 20.510 | 275.2 | 0.0 | 2.726 | 17.940 | 1.773 | 99.3 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:10:00 | 5.896 | 5.872 | 5.318 | 4.967 | 4.892 | 4.778 | 0.573 | 0.528 | 0.640 | 0.635 | ... | 14.600 | 275.2 | 0.0 | 3.750 | 15.530 | 1.641 | 100.0 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:20:00 | 4.897 | 4.877 | 4.583 | 4.469 | 4.272 | 4.250 | 0.759 | 0.696 | 0.731 | 0.676 | ... | 14.260 | 275.2 | 0.0 | 15.830 | 17.620 | 1.510 | 100.0 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:30:00 | 4.830 | 4.859 | 4.135 | 4.106 | 3.775 | 3.751 | 0.797 | 0.739 | 0.871 | 0.831 | ... | 6.807 | 275.2 | 0.0 | 45.140 | 8.820 | 1.541 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 02:40:00 | 4.728 | 4.716 | 4.353 | 4.322 | 4.006 | 4.001 | 0.510 | 0.433 | 0.470 | 0.396 | ... | 7.454 | 275.2 | 0.0 | 36.230 | 7.463 | 1.551 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 02:50:00 | 4.207 | 4.221 | 3.646 | 3.563 | 3.105 | 3.008 | 1.211 | 1.228 | 1.162 | 1.235 | ... | 12.380 | 275.2 | 0.0 | 9.620 | 20.540 | 1.398 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 03:00:00 | 4.315 | 4.267 | 3.923 | 3.754 | 3.590 | 3.515 | 0.569 | 0.511 | 0.529 | 0.516 | ... | 11.910 | 275.2 | 0.0 | 5.029 | 10.810 | 1.470 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 03:10:00 | 5.976 | 5.952 | 5.513 | 5.359 | 4.838 | 4.746 | 0.717 | 0.667 | 0.839 | 0.935 | ... | 9.510 | 275.2 | 0.0 | 6.449 | 14.240 | 1.530 | 100.0 | 967.0 | 0.0 | 12.68 |
2017-01-01 03:20:00 | 6.539 | 6.550 | 5.985 | 5.969 | 5.471 | 5.467 | 0.803 | 0.761 | 0.745 | 0.741 | ... | 4.772 | 275.2 | 0.0 | 10.840 | 11.510 | 1.439 | 100.0 | 967.0 | 0.0 | 12.68 |
2017-01-01 03:30:00 | 7.523 | 7.566 | 7.091 | 7.106 | 6.509 | 6.521 | 0.549 | 0.484 | 0.576 | 0.516 | ... | 2.797 | 275.2 | 0.0 | 12.380 | 4.930 | 1.500 | 100.0 | 967.0 | 0.0 | 12.68 |
2017-01-01 03:40:00 | 7.904 | 7.953 | 7.640 | 7.637 | 7.220 | 7.215 | 0.497 | 0.428 | 0.656 | 0.616 | ... | 2.781 | 275.2 | 0.0 | 18.510 | 5.389 | 1.570 | 100.0 | 967.0 | 0.0 | 12.67 |
2017-01-01 03:50:00 | 7.621 | 7.626 | 7.261 | 7.189 | 6.824 | 6.801 | 0.683 | 0.637 | 0.750 | 0.725 | ... | 9.960 | 275.2 | 0.0 | 14.650 | 8.850 | 1.520 | 99.9 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:00:00 | 7.791 | 7.850 | 7.277 | 7.276 | 6.593 | 6.618 | 1.116 | 1.056 | 1.153 | 1.108 | ... | 6.700 | 275.2 | 0.0 | 11.620 | 11.890 | 1.379 | 100.0 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:10:00 | 8.190 | 8.230 | 7.611 | 7.636 | 7.090 | 7.111 | 1.079 | 1.003 | 1.251 | 1.249 | ... | 8.580 | 275.2 | 0.0 | 13.020 | 11.540 | 1.288 | 100.0 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:20:00 | 8.200 | 8.240 | 7.179 | 7.210 | 6.591 | 6.635 | 1.491 | 1.428 | 1.501 | 1.466 | ... | 8.820 | 275.2 | 0.0 | 16.440 | 11.740 | 1.136 | 98.5 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:30:00 | 8.290 | 8.370 | 7.685 | 7.707 | 6.911 | 6.920 | 0.996 | 0.948 | 1.137 | 1.117 | ... | 9.960 | 275.2 | 0.0 | 15.710 | 14.230 | 1.045 | 97.5 | 968.0 | 0.0 | 12.67 |
2017-01-01 04:40:00 | 8.230 | 8.290 | 7.376 | 7.299 | 6.835 | 6.849 | 1.071 | 1.033 | 1.231 | 1.126 | ... | 12.660 | 275.2 | 0.0 | 10.550 | 13.360 | 0.792 | 96.0 | 968.0 | 0.0 | 12.67 |
2017-01-01 04:50:00 | 9.600 | 9.660 | 8.890 | 8.850 | 8.170 | 8.190 | 1.075 | 1.040 | 1.033 | 1.094 | ... | 6.267 | 275.2 | 0.0 | 8.210 | 15.310 | 0.468 | 95.4 | 968.0 | 0.0 | 12.67 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2017-02-01 19:00:00 | 9.980 | 9.970 | 7.821 | 8.970 | 7.269 | 8.350 | 1.556 | 1.471 | 1.402 | 1.335 | ... | 5.712 | 275.2 | 0.0 | 170.500 | 6.764 | 4.370 | 98.8 | 947.0 | 0.0 | 12.98 |
2017-02-01 19:10:00 | 7.264 | 7.333 | 5.912 | 6.739 | 5.534 | 6.465 | 1.459 | 1.326 | 1.319 | 1.074 | ... | 8.560 | 275.2 | 0.0 | 170.900 | 7.869 | 4.512 | 97.3 | 946.0 | 0.0 | 12.98 |
2017-02-01 19:20:00 | 8.210 | 8.270 | 6.920 | 7.266 | 6.077 | 6.393 | 1.875 | 1.738 | 1.835 | 1.572 | ... | 10.950 | 275.2 | 0.0 | 160.700 | 11.660 | 4.814 | 96.8 | 947.0 | 0.0 | 12.97 |
2017-02-01 19:30:00 | 11.040 | 10.980 | 10.010 | 9.960 | 9.180 | 9.180 | 1.520 | 1.435 | 1.551 | 1.421 | ... | 5.745 | 275.2 | 0.0 | 156.000 | 6.775 | 5.138 | 95.4 | 946.0 | 0.0 | 12.96 |
2017-02-01 19:40:00 | 12.240 | 12.100 | 11.570 | 11.390 | 11.040 | 10.870 | 1.440 | 1.362 | 1.444 | 1.332 | ... | 5.474 | 275.2 | 0.0 | 153.500 | 6.161 | 5.562 | 95.5 | 946.0 | 0.0 | 12.96 |
2017-02-01 19:50:00 | 13.340 | 13.250 | 12.650 | 12.490 | 11.990 | 11.830 | 1.632 | 1.571 | 1.846 | 1.659 | ... | 5.866 | 275.2 | 0.0 | 152.800 | 6.037 | 5.583 | 94.3 | 946.0 | 0.0 | 12.95 |
2017-02-01 20:00:00 | 14.150 | 14.070 | 13.570 | 13.280 | 12.890 | 12.670 | 1.196 | 1.103 | 1.161 | 1.076 | ... | 5.031 | 275.2 | 0.0 | 150.800 | 5.244 | 5.623 | 94.8 | 945.0 | 0.0 | 12.94 |
2017-02-01 20:10:00 | 15.540 | 15.460 | 14.930 | 14.700 | 14.450 | 14.280 | 1.321 | 1.245 | 1.497 | 1.374 | ... | 4.250 | 275.2 | 0.0 | 148.200 | 5.148 | 5.461 | 95.1 | 945.0 | 0.0 | 12.93 |
2017-02-01 20:20:00 | 14.870 | 14.860 | 14.280 | 14.060 | 13.850 | 13.650 | 1.292 | 1.204 | 1.556 | 1.457 | ... | 3.911 | 275.2 | 0.0 | 146.800 | 4.782 | 5.421 | 97.1 | 945.0 | 0.0 | 12.93 |
2017-02-01 20:30:00 | 15.220 | 15.220 | 14.920 | 14.750 | 14.340 | 14.190 | 1.244 | 1.174 | 1.198 | 1.143 | ... | 4.193 | 275.2 | 0.0 | 146.100 | 4.854 | 5.502 | 97.8 | 945.0 | 0.0 | 12.93 |
2017-02-01 20:40:00 | 14.510 | 14.500 | 14.230 | 14.030 | 13.760 | 13.600 | 1.362 | 1.273 | 1.459 | 1.373 | ... | 4.882 | 275.2 | 0.0 | 148.500 | 4.689 | 5.542 | 98.3 | 945.0 | 0.0 | 12.92 |
2017-02-01 20:50:00 | 13.570 | 13.510 | 13.110 | 12.910 | 12.690 | 12.550 | 1.465 | 1.380 | 1.592 | 1.458 | ... | 5.153 | 275.2 | 0.0 | 152.800 | 5.701 | 5.461 | 99.1 | 945.0 | 0.1 | 12.92 |
2017-02-01 21:00:00 | 13.200 | 13.120 | 12.100 | 12.080 | 11.540 | 11.540 | 1.683 | 1.569 | 1.678 | 1.478 | ... | 6.637 | 275.2 | 0.0 | 155.700 | 6.759 | 5.592 | 100.0 | 945.0 | 0.1 | 12.91 |
2017-02-01 21:10:00 | 12.780 | 12.840 | 11.880 | 11.990 | 11.040 | 11.270 | 2.181 | 2.064 | 2.074 | 1.846 | ... | 6.751 | 275.2 | 0.0 | 156.200 | 8.080 | 5.572 | 100.0 | 945.0 | 0.1 | 12.91 |
2017-02-01 21:20:00 | 13.230 | 13.190 | 13.010 | 12.900 | 12.680 | 12.670 | 1.995 | 1.907 | 1.828 | 1.734 | ... | 7.287 | 275.2 | 0.0 | 153.200 | 6.439 | 5.602 | 100.0 | 945.0 | 0.1 | 12.91 |
2017-02-01 21:30:00 | 12.160 | 12.210 | 11.190 | 11.450 | 10.670 | 10.940 | 2.316 | 2.182 | 2.726 | 2.271 | ... | 8.080 | 275.2 | 0.0 | 158.100 | 8.290 | 5.592 | 100.0 | 945.0 | 0.2 | 12.91 |
2017-02-01 21:40:00 | 11.400 | 11.690 | 9.840 | 10.690 | 9.060 | 9.860 | 1.785 | 1.759 | 1.655 | 1.527 | ... | 8.090 | 275.2 | 0.0 | 163.600 | 9.410 | 5.592 | 100.0 | 944.0 | 0.2 | 12.90 |
2017-02-01 21:50:00 | 11.530 | 11.770 | 10.270 | 10.910 | 9.930 | 10.460 | 2.281 | 2.186 | 2.116 | 2.102 | ... | 9.460 | 275.2 | 0.0 | 159.600 | 11.100 | 5.673 | 100.0 | 945.0 | 0.2 | 12.90 |
2017-02-01 22:00:00 | 11.940 | 12.190 | 10.120 | 11.330 | 9.520 | 11.000 | 2.337 | 2.232 | 2.613 | 2.416 | ... | 8.220 | 275.2 | 0.0 | 168.200 | 7.792 | 5.531 | 100.0 | 944.0 | 0.2 | 12.89 |
2017-02-01 22:10:00 | 11.850 | 12.060 | 9.970 | 11.290 | 9.230 | 10.570 | 1.937 | 1.833 | 2.190 | 1.828 | ... | 8.860 | 275.2 | 0.0 | 170.700 | 8.850 | 5.421 | 100.0 | 944.0 | 0.1 | 12.89 |
2017-02-01 22:20:00 | 10.240 | 10.390 | 8.220 | 9.700 | 7.887 | 9.290 | 1.740 | 1.657 | 1.474 | 1.462 | ... | 8.550 | 275.2 | 0.0 | 176.200 | 8.810 | 5.279 | 100.0 | 944.0 | 0.0 | 12.89 |
2017-02-01 22:30:00 | 11.000 | 11.050 | 8.270 | 10.050 | 8.110 | 9.410 | 1.421 | 1.318 | 1.443 | 1.579 | ... | 6.332 | 275.2 | 0.0 | 181.800 | 7.599 | 5.309 | 100.0 | 944.0 | 0.0 | 12.89 |
2017-02-01 22:40:00 | 11.900 | 12.000 | 9.100 | 11.060 | 8.720 | 10.270 | 1.561 | 1.454 | 1.584 | 1.438 | ... | 6.540 | 275.2 | 0.0 | 175.800 | 8.180 | 5.411 | 100.0 | 944.0 | 0.0 | 12.89 |
2017-02-01 22:50:00 | 11.160 | 11.250 | 8.280 | 10.310 | 7.959 | 9.430 | 2.197 | 2.084 | 1.699 | 1.934 | ... | 6.301 | 275.2 | 0.0 | 176.700 | 8.300 | 5.481 | 100.0 | 944.0 | 0.0 | 12.88 |
2017-02-01 23:00:00 | 10.580 | 10.760 | 8.310 | 9.800 | 8.080 | 9.270 | 1.974 | 1.819 | 1.733 | 1.689 | ... | 8.480 | 275.2 | 0.0 | 172.100 | 8.700 | 5.552 | 100.0 | 944.0 | 0.1 | 12.88 |
2017-02-01 23:10:00 | 11.190 | 11.410 | 8.710 | 10.480 | 8.240 | 9.790 | 1.821 | 1.691 | 1.608 | 1.472 | ... | 7.089 | 275.2 | 0.0 | 172.200 | 6.555 | 5.491 | 100.0 | 944.0 | 0.0 | 12.88 |
2017-02-01 23:20:00 | 11.580 | 11.750 | 9.150 | 10.820 | 8.840 | 10.120 | 2.490 | 2.315 | 2.191 | 2.361 | ... | 7.986 | 275.2 | 0.0 | 180.000 | 8.520 | 5.542 | 100.0 | 944.0 | 0.1 | 12.88 |
2017-02-01 23:30:00 | 14.320 | 14.490 | 10.770 | 13.280 | 10.430 | 12.200 | 2.221 | 1.981 | 1.867 | 1.856 | ... | 6.347 | 275.2 | 0.0 | 177.700 | 7.901 | 5.602 | 100.0 | 944.0 | 0.0 | 12.88 |
2017-02-01 23:40:00 | 12.870 | 12.860 | 10.650 | 12.010 | 10.250 | 11.320 | 2.060 | 1.905 | 1.994 | 1.764 | ... | 7.929 | 275.2 | 0.0 | 185.400 | 9.620 | 5.643 | 100.0 | 944.0 | 0.0 | 12.88 |
2017-02-01 23:50:00 | 13.330 | 13.440 | 10.920 | 12.630 | 10.640 | 11.770 | 2.425 | 2.258 | 2.454 | 2.119 | ... | 7.456 | 275.2 | 0.0 | 183.100 | 8.470 | 5.643 | 100.0 | 944.0 | 0.2 | 12.88 |
4608 rows × 29 columns
To select all the data points from a specific date, i.e. 2017-01-01, to the end of the series you can leave the area after the ‘:’ empty.
[8]:
data['2017-01-01':]
[8]:
Spd80mN | Spd80mS | Spd60mN | Spd60mS | Spd40mN | Spd40mS | Spd80mNStd | Spd80mSStd | Spd60mNStd | Spd60mSStd | ... | Dir78mSStd | Dir58mS | Dir58mSStd | Dir38mS | Dir38mSStd | T2m | RH2m | P2m | PrcpTot | BattMin | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | |||||||||||||||||||||
2017-01-01 00:00:00 | 5.876 | 5.858 | 5.747 | 5.744 | 5.605 | 5.558 | 1.160 | 1.109 | 1.186 | 1.158 | ... | 7.025 | 275.2 | 0.0 | 324.900 | 6.451 | 3.077 | 99.7 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:10:00 | 5.911 | 5.898 | 5.539 | 5.621 | 5.324 | 5.345 | 1.114 | 1.079 | 1.005 | 0.977 | ... | 7.498 | 275.2 | 0.0 | 319.600 | 9.160 | 2.915 | 99.8 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:20:00 | 7.004 | 6.967 | 6.741 | 6.797 | 6.645 | 6.634 | 1.038 | 0.998 | 1.042 | 1.033 | ... | 6.283 | 275.2 | 0.0 | 316.500 | 7.228 | 2.865 | 99.8 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:30:00 | 7.079 | 7.067 | 6.832 | 6.881 | 6.792 | 6.801 | 0.705 | 0.667 | 0.707 | 0.665 | ... | 4.661 | 275.2 | 0.0 | 319.100 | 5.279 | 2.693 | 97.8 | 966.0 | 0.0 | 12.71 |
2017-01-01 00:40:00 | 6.796 | 6.765 | 6.684 | 6.742 | 6.658 | 6.685 | 0.794 | 0.785 | 0.812 | 0.792 | ... | 5.036 | 275.2 | 0.0 | 317.300 | 6.070 | 2.471 | 98.4 | 967.0 | 0.0 | 12.71 |
2017-01-01 00:50:00 | 8.380 | 8.350 | 8.070 | 8.170 | 7.861 | 7.915 | 0.999 | 0.949 | 0.920 | 0.871 | ... | 5.734 | 275.2 | 0.0 | 314.400 | 5.881 | 2.329 | 99.1 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:00:00 | 8.910 | 8.870 | 8.310 | 8.400 | 7.992 | 7.991 | 0.751 | 0.717 | 0.809 | 0.775 | ... | 3.719 | 275.2 | 0.0 | 315.900 | 4.887 | 2.137 | 98.5 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:10:00 | 8.490 | 8.460 | 8.010 | 8.050 | 7.507 | 7.526 | 0.720 | 0.665 | 0.779 | 0.756 | ... | 4.544 | 275.2 | 0.0 | 323.500 | 5.816 | 2.006 | 99.0 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:20:00 | 7.116 | 7.096 | 6.755 | 6.739 | 6.410 | 6.354 | 0.719 | 0.678 | 0.741 | 0.700 | ... | 5.676 | 275.2 | 0.0 | 326.100 | 5.782 | 1.783 | 98.9 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:30:00 | 8.060 | 8.070 | 7.415 | 7.446 | 6.812 | 6.814 | 0.867 | 0.835 | 0.912 | 0.827 | ... | 5.352 | 275.2 | 0.0 | 323.600 | 6.129 | 1.742 | 99.6 | 966.0 | 0.0 | 12.70 |
2017-01-01 01:40:00 | 9.150 | 9.170 | 8.480 | 8.300 | 7.883 | 7.721 | 0.738 | 0.685 | 0.789 | 0.750 | ... | 5.377 | 275.2 | 0.0 | 330.600 | 6.721 | 1.763 | 99.8 | 966.0 | 0.1 | 12.70 |
2017-01-01 01:50:00 | 7.837 | 7.820 | 7.132 | 6.473 | 6.608 | 6.100 | 0.837 | 0.834 | 1.013 | 1.140 | ... | 14.100 | 275.2 | 0.0 | 339.700 | 6.033 | 1.783 | 99.3 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:00:00 | 6.234 | 6.149 | 5.446 | 4.847 | 4.863 | 4.624 | 1.133 | 1.067 | 0.962 | 0.795 | ... | 20.510 | 275.2 | 0.0 | 2.726 | 17.940 | 1.773 | 99.3 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:10:00 | 5.896 | 5.872 | 5.318 | 4.967 | 4.892 | 4.778 | 0.573 | 0.528 | 0.640 | 0.635 | ... | 14.600 | 275.2 | 0.0 | 3.750 | 15.530 | 1.641 | 100.0 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:20:00 | 4.897 | 4.877 | 4.583 | 4.469 | 4.272 | 4.250 | 0.759 | 0.696 | 0.731 | 0.676 | ... | 14.260 | 275.2 | 0.0 | 15.830 | 17.620 | 1.510 | 100.0 | 966.0 | 0.0 | 12.69 |
2017-01-01 02:30:00 | 4.830 | 4.859 | 4.135 | 4.106 | 3.775 | 3.751 | 0.797 | 0.739 | 0.871 | 0.831 | ... | 6.807 | 275.2 | 0.0 | 45.140 | 8.820 | 1.541 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 02:40:00 | 4.728 | 4.716 | 4.353 | 4.322 | 4.006 | 4.001 | 0.510 | 0.433 | 0.470 | 0.396 | ... | 7.454 | 275.2 | 0.0 | 36.230 | 7.463 | 1.551 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 02:50:00 | 4.207 | 4.221 | 3.646 | 3.563 | 3.105 | 3.008 | 1.211 | 1.228 | 1.162 | 1.235 | ... | 12.380 | 275.2 | 0.0 | 9.620 | 20.540 | 1.398 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 03:00:00 | 4.315 | 4.267 | 3.923 | 3.754 | 3.590 | 3.515 | 0.569 | 0.511 | 0.529 | 0.516 | ... | 11.910 | 275.2 | 0.0 | 5.029 | 10.810 | 1.470 | 100.0 | 967.0 | 0.0 | 12.69 |
2017-01-01 03:10:00 | 5.976 | 5.952 | 5.513 | 5.359 | 4.838 | 4.746 | 0.717 | 0.667 | 0.839 | 0.935 | ... | 9.510 | 275.2 | 0.0 | 6.449 | 14.240 | 1.530 | 100.0 | 967.0 | 0.0 | 12.68 |
2017-01-01 03:20:00 | 6.539 | 6.550 | 5.985 | 5.969 | 5.471 | 5.467 | 0.803 | 0.761 | 0.745 | 0.741 | ... | 4.772 | 275.2 | 0.0 | 10.840 | 11.510 | 1.439 | 100.0 | 967.0 | 0.0 | 12.68 |
2017-01-01 03:30:00 | 7.523 | 7.566 | 7.091 | 7.106 | 6.509 | 6.521 | 0.549 | 0.484 | 0.576 | 0.516 | ... | 2.797 | 275.2 | 0.0 | 12.380 | 4.930 | 1.500 | 100.0 | 967.0 | 0.0 | 12.68 |
2017-01-01 03:40:00 | 7.904 | 7.953 | 7.640 | 7.637 | 7.220 | 7.215 | 0.497 | 0.428 | 0.656 | 0.616 | ... | 2.781 | 275.2 | 0.0 | 18.510 | 5.389 | 1.570 | 100.0 | 967.0 | 0.0 | 12.67 |
2017-01-01 03:50:00 | 7.621 | 7.626 | 7.261 | 7.189 | 6.824 | 6.801 | 0.683 | 0.637 | 0.750 | 0.725 | ... | 9.960 | 275.2 | 0.0 | 14.650 | 8.850 | 1.520 | 99.9 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:00:00 | 7.791 | 7.850 | 7.277 | 7.276 | 6.593 | 6.618 | 1.116 | 1.056 | 1.153 | 1.108 | ... | 6.700 | 275.2 | 0.0 | 11.620 | 11.890 | 1.379 | 100.0 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:10:00 | 8.190 | 8.230 | 7.611 | 7.636 | 7.090 | 7.111 | 1.079 | 1.003 | 1.251 | 1.249 | ... | 8.580 | 275.2 | 0.0 | 13.020 | 11.540 | 1.288 | 100.0 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:20:00 | 8.200 | 8.240 | 7.179 | 7.210 | 6.591 | 6.635 | 1.491 | 1.428 | 1.501 | 1.466 | ... | 8.820 | 275.2 | 0.0 | 16.440 | 11.740 | 1.136 | 98.5 | 967.0 | 0.0 | 12.67 |
2017-01-01 04:30:00 | 8.290 | 8.370 | 7.685 | 7.707 | 6.911 | 6.920 | 0.996 | 0.948 | 1.137 | 1.117 | ... | 9.960 | 275.2 | 0.0 | 15.710 | 14.230 | 1.045 | 97.5 | 968.0 | 0.0 | 12.67 |
2017-01-01 04:40:00 | 8.230 | 8.290 | 7.376 | 7.299 | 6.835 | 6.849 | 1.071 | 1.033 | 1.231 | 1.126 | ... | 12.660 | 275.2 | 0.0 | 10.550 | 13.360 | 0.792 | 96.0 | 968.0 | 0.0 | 12.67 |
2017-01-01 04:50:00 | 9.600 | 9.660 | 8.890 | 8.850 | 8.170 | 8.190 | 1.075 | 1.040 | 1.033 | 1.094 | ... | 6.267 | 275.2 | 0.0 | 8.210 | 15.310 | 0.468 | 95.4 | 968.0 | 0.0 | 12.67 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2017-11-23 06:00:00 | 10.620 | 0.000 | 10.420 | 10.370 | 10.110 | 10.030 | 0.857 | 0.000 | 0.803 | 0.752 | ... | 0.000 | 275.2 | 0.0 | 257.200 | 4.303 | 0.589 | 100.0 | 940.0 | 0.1 | 12.71 |
2017-11-23 06:10:00 | 10.720 | 0.000 | 10.670 | 10.600 | 10.470 | 10.380 | 1.027 | 0.000 | 0.938 | 0.919 | ... | 0.000 | 275.2 | 0.0 | 258.700 | 4.351 | 0.701 | 100.0 | 940.0 | 0.0 | 12.71 |
2017-11-23 06:20:00 | 10.940 | 0.000 | 10.650 | 10.600 | 10.290 | 10.210 | 0.708 | 0.000 | 0.823 | 0.778 | ... | 0.000 | 275.2 | 0.0 | 257.700 | 3.923 | 0.761 | 99.5 | 940.0 | 0.0 | 12.71 |
2017-11-23 06:30:00 | 10.270 | 0.000 | 10.130 | 9.960 | 9.910 | 9.790 | 0.800 | 0.000 | 0.865 | 0.808 | ... | 0.000 | 275.2 | 0.0 | 251.600 | 3.722 | 0.792 | 98.0 | 940.0 | 0.0 | 12.71 |
2017-11-23 06:40:00 | 8.180 | 0.000 | 7.985 | 7.811 | 7.649 | 7.519 | 1.167 | 0.000 | 1.239 | 1.199 | ... | 0.000 | 275.2 | 0.0 | 242.800 | 4.858 | 0.518 | 98.2 | 941.0 | 0.0 | 12.71 |
2017-11-23 06:50:00 | 8.870 | 0.000 | 8.570 | 8.330 | 8.020 | 7.873 | 0.676 | 0.000 | 0.582 | 0.534 | ... | 0.000 | 275.2 | 0.0 | 232.900 | 3.426 | 0.782 | 99.9 | 941.0 | 0.0 | 12.71 |
2017-11-23 07:00:00 | 9.300 | 0.000 | 8.950 | 8.670 | 8.590 | 8.400 | 0.933 | 0.000 | 0.994 | 0.937 | ... | 0.000 | 275.2 | 0.0 | 229.300 | 4.575 | 0.761 | 99.5 | 941.0 | 0.0 | 12.71 |
2017-11-23 07:10:00 | 8.130 | 0.000 | 7.863 | 7.592 | 7.283 | 7.094 | 0.823 | 0.000 | 0.837 | 0.794 | ... | 0.000 | 275.2 | 0.0 | 225.100 | 4.478 | 0.721 | 99.9 | 941.0 | 0.0 | 12.67 |
2017-11-23 07:20:00 | 8.910 | 0.000 | 8.500 | 8.250 | 8.020 | 7.864 | 1.063 | 0.000 | 0.977 | 0.929 | ... | 0.000 | 275.2 | 0.0 | 226.700 | 5.236 | 0.751 | 100.0 | 941.0 | 0.0 | 12.66 |
2017-11-23 07:30:00 | 9.610 | 0.000 | 9.260 | 8.980 | 8.630 | 8.470 | 0.942 | 0.000 | 0.923 | 0.860 | ... | 0.000 | 275.2 | 0.0 | 228.100 | 5.127 | 0.670 | 100.0 | 941.0 | 0.0 | 12.66 |
2017-11-23 07:40:00 | 10.900 | 0.000 | 10.310 | 10.050 | 9.680 | 9.490 | 1.268 | 0.000 | 1.170 | 1.111 | ... | 0.000 | 275.2 | 0.0 | 232.700 | 5.563 | 0.570 | 100.0 | 941.0 | 0.0 | 12.67 |
2017-11-23 07:50:00 | 11.560 | 0.000 | 11.080 | 10.790 | 10.400 | 10.190 | 1.022 | 0.000 | 1.114 | 1.067 | ... | 0.000 | 275.2 | 0.0 | 238.600 | 4.363 | 0.751 | 100.0 | 941.0 | 0.0 | 12.70 |
2017-11-23 08:00:00 | 11.730 | 0.000 | 11.290 | 11.030 | 10.690 | 10.490 | 0.842 | 0.000 | 0.834 | 0.759 | ... | 0.000 | 275.2 | 0.0 | 237.400 | 3.395 | 0.771 | 100.0 | 941.0 | 0.0 | 12.70 |
2017-11-23 08:10:00 | 11.700 | 0.000 | 11.420 | 11.130 | 11.030 | 10.830 | 0.764 | 0.000 | 0.727 | 0.673 | ... | 0.000 | 275.2 | 0.0 | 235.900 | 3.391 | 0.863 | 99.7 | 941.0 | 0.0 | 12.66 |
2017-11-23 08:20:00 | 12.270 | 0.000 | 12.020 | 11.760 | 11.510 | 11.330 | 0.774 | 0.000 | 0.800 | 0.755 | ... | 0.000 | 275.2 | 0.0 | 237.000 | 3.657 | 0.842 | 99.5 | 941.0 | 0.0 | 12.66 |
2017-11-23 08:30:00 | 11.510 | 0.000 | 11.260 | 10.990 | 10.740 | 10.530 | 0.956 | 0.000 | 0.903 | 0.857 | ... | 0.000 | 275.2 | 0.0 | 234.600 | 3.988 | 0.701 | 99.3 | 942.0 | 0.0 | 12.65 |
2017-11-23 08:40:00 | 11.380 | 0.000 | 10.850 | 10.520 | 10.310 | 10.090 | 0.962 | 0.000 | 0.867 | 0.840 | ... | 0.000 | 275.2 | 0.0 | 235.000 | 4.448 | 0.751 | 99.7 | 942.0 | 0.0 | 12.68 |
2017-11-23 08:50:00 | 10.420 | 0.000 | 10.090 | 9.810 | 9.590 | 9.380 | 1.034 | 0.000 | 1.141 | 1.111 | ... | 0.000 | 275.2 | 0.0 | 223.700 | 7.394 | 0.680 | 99.7 | 942.0 | 0.0 | 12.69 |
2017-11-23 09:00:00 | 9.050 | 0.000 | 8.530 | 8.270 | 7.696 | 7.503 | 0.668 | 0.000 | 0.763 | 0.681 | ... | 0.000 | 275.2 | 0.0 | 211.600 | 7.172 | 0.812 | 100.0 | 942.0 | 0.0 | 12.70 |
2017-11-23 09:10:00 | 7.484 | 0.000 | 7.231 | 6.956 | 6.596 | 6.404 | 1.009 | 0.000 | 0.868 | 0.811 | ... | 0.000 | 275.2 | 0.0 | 213.000 | 7.851 | 0.771 | 98.4 | 942.0 | 0.0 | 12.69 |
2017-11-23 09:20:00 | 7.228 | 0.000 | 6.903 | 6.691 | 6.273 | 6.089 | 0.756 | 0.000 | 0.841 | 0.753 | ... | 0.000 | 275.2 | 0.0 | 209.100 | 7.654 | 0.549 | 99.0 | 943.0 | 0.0 | 12.70 |
2017-11-23 09:30:00 | 7.740 | 0.000 | 7.359 | 7.147 | 6.889 | 6.775 | 0.634 | 0.000 | 0.618 | 0.586 | ... | 0.000 | 275.2 | 0.0 | 221.000 | 6.078 | 0.711 | 99.6 | 943.0 | 0.0 | 12.52 |
2017-11-23 09:40:00 | 8.380 | 0.000 | 7.900 | 7.675 | 7.190 | 7.068 | 0.822 | 0.000 | 0.881 | 0.798 | ... | 0.000 | 275.2 | 0.0 | 221.900 | 4.969 | 0.651 | 98.8 | 943.0 | 0.0 | 12.73 |
2017-11-23 09:50:00 | 9.870 | 0.000 | 9.250 | 8.970 | 8.450 | 8.250 | 0.954 | 0.000 | 0.878 | 0.805 | ... | 0.000 | 275.2 | 0.0 | 220.200 | 5.269 | 0.873 | 99.8 | 943.0 | 0.0 | 12.89 |
2017-11-23 10:00:00 | 9.800 | 0.000 | 9.340 | 9.070 | 8.630 | 8.450 | 1.170 | 0.000 | 1.136 | 1.076 | ... | 0.000 | 275.2 | 0.0 | 221.900 | 5.125 | 0.731 | 98.6 | 943.0 | 0.0 | 12.94 |
2017-11-23 10:10:00 | 10.480 | 0.000 | 10.190 | 9.890 | 9.590 | 9.420 | 0.720 | 0.000 | 0.733 | 0.668 | ... | 0.000 | 275.2 | 0.0 | 222.200 | 4.111 | 0.943 | 99.7 | 943.0 | 0.0 | 13.02 |
2017-11-23 10:20:00 | 9.390 | 0.000 | 9.120 | 8.850 | 8.520 | 8.340 | 0.659 | 0.000 | 0.734 | 0.651 | ... | 0.000 | 275.2 | 0.0 | 218.400 | 4.817 | 0.792 | 98.6 | 943.0 | 0.0 | 13.69 |
2017-11-23 10:30:00 | 9.140 | 0.000 | 8.700 | 8.450 | 8.030 | 7.875 | 0.689 | 0.000 | 0.821 | 0.732 | ... | 0.000 | 275.2 | 0.0 | 216.000 | 5.784 | 0.802 | 100.0 | 943.0 | 0.0 | 13.86 |
2017-11-23 10:40:00 | 7.927 | 0.000 | 7.383 | 7.159 | 6.811 | 6.668 | 0.817 | 0.000 | 0.769 | 0.692 | ... | 0.000 | 275.2 | 0.0 | 219.500 | 5.051 | 0.883 | 100.0 | 943.0 | 0.0 | 13.80 |
2017-11-23 10:50:00 | 7.120 | 0.000 | 6.617 | 6.404 | 5.865 | 5.749 | 0.537 | 0.000 | 0.534 | 0.450 | ... | 0.000 | 275.2 | 0.0 | 222.400 | 4.902 | 0.802 | 100.0 | 944.0 | 0.0 | 13.71 |
47010 rows × 29 columns
To select all the data points from the start to a specific date, i.e. 2017-01-01, you can leave the area before the ‘:’ empty.
[9]:
data[:'2017-01-01']
[9]:
Spd80mN | Spd80mS | Spd60mN | Spd60mS | Spd40mN | Spd40mS | Spd80mNStd | Spd80mSStd | Spd60mNStd | Spd60mSStd | ... | Dir78mSStd | Dir58mS | Dir58mSStd | Dir38mS | Dir38mSStd | T2m | RH2m | P2m | PrcpTot | BattMin | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | |||||||||||||||||||||
2016-01-09 15:30:00 | 8.370 | 7.911 | 8.160 | 7.849 | 7.857 | 7.626 | 1.240 | 1.075 | 1.060 | 0.947 | ... | 6.100 | 110.10 | 6.009 | 112.200 | 5.724 | 0.711 | 100.0 | 935.0 | 0.0 | 12.94 |
2016-01-09 15:40:00 | 8.250 | 7.961 | 8.100 | 7.884 | 7.952 | 7.840 | 0.897 | 0.875 | 0.900 | 0.855 | ... | 5.114 | 110.90 | 4.702 | 109.800 | 5.628 | 0.630 | 100.0 | 935.0 | 0.0 | 12.95 |
2016-01-09 17:00:00 | 7.652 | 7.545 | 7.671 | 7.551 | 7.531 | 7.457 | 0.756 | 0.703 | 0.797 | 0.749 | ... | 4.172 | 113.10 | 3.447 | 111.800 | 4.016 | 1.126 | 100.0 | 934.0 | 0.0 | 12.75 |
2016-01-09 17:10:00 | 7.382 | 7.325 | 6.818 | 6.689 | 6.252 | 6.174 | 0.844 | 0.810 | 0.897 | 0.875 | ... | 4.680 | 118.80 | 5.107 | 115.600 | 5.189 | 0.954 | 100.0 | 934.0 | 0.0 | 12.71 |
2016-01-09 17:20:00 | 7.977 | 7.791 | 8.110 | 7.915 | 8.140 | 7.974 | 0.556 | 0.528 | 0.562 | 0.524 | ... | 3.123 | 115.90 | 2.960 | 113.600 | 3.540 | 0.863 | 100.0 | 934.0 | 0.0 | 12.69 |
2016-01-09 17:30:00 | 8.340 | 8.160 | 8.370 | 8.170 | 8.330 | 8.180 | 0.676 | 0.607 | 0.756 | 0.708 | ... | 3.260 | 117.20 | 3.600 | 117.400 | 4.526 | 0.731 | 100.0 | 934.0 | 0.0 | 12.67 |
2016-01-09 17:40:00 | 8.130 | 7.929 | 8.090 | 7.895 | 7.972 | 7.788 | 0.557 | 0.507 | 0.534 | 0.498 | ... | 3.677 | 115.90 | 3.371 | 115.900 | 3.515 | 0.852 | 100.0 | 933.0 | 0.0 | 12.68 |
2016-01-09 17:50:00 | 7.480 | 7.283 | 7.706 | 7.486 | 7.649 | 7.481 | 0.588 | 0.526 | 0.590 | 0.529 | ... | 3.500 | 119.90 | 3.265 | 118.900 | 3.322 | 0.771 | 100.0 | 933.0 | 0.0 | 12.67 |
2016-01-09 18:00:00 | 7.554 | 7.452 | 7.484 | 7.359 | 7.578 | 7.456 | 0.734 | 0.681 | 0.631 | 0.573 | ... | 3.061 | 113.90 | 2.663 | 111.400 | 2.789 | 0.913 | 100.0 | 933.0 | 0.0 | 12.65 |
2016-01-09 18:10:00 | 8.220 | 8.070 | 8.080 | 7.888 | 7.791 | 7.639 | 0.743 | 0.724 | 0.800 | 0.757 | ... | 3.427 | 115.10 | 4.055 | 113.800 | 4.834 | 0.832 | 100.0 | 933.0 | 0.0 | 12.62 |
2016-01-09 18:20:00 | 9.420 | 9.270 | 9.660 | 9.470 | 9.570 | 9.450 | 0.482 | 0.438 | 0.566 | 0.522 | ... | 2.431 | 119.50 | 2.454 | 117.400 | 3.017 | 0.549 | 100.0 | 933.0 | 0.0 | 12.61 |
2016-01-09 18:30:00 | 9.910 | 9.810 | 9.610 | 9.480 | 8.940 | 8.840 | 0.568 | 0.513 | 0.698 | 0.648 | ... | 3.272 | 114.90 | 3.271 | 113.200 | 4.454 | 0.529 | 100.0 | 933.0 | 0.0 | 12.60 |
2016-01-09 18:40:00 | 10.390 | 10.340 | 10.260 | 10.110 | 9.840 | 9.760 | 0.683 | 0.640 | 0.710 | 0.696 | ... | 4.405 | 110.90 | 4.308 | 109.300 | 4.776 | 0.589 | 100.0 | 933.0 | 0.0 | 12.60 |
2016-01-09 18:50:00 | 10.360 | 10.320 | 10.320 | 10.230 | 9.830 | 9.820 | 0.840 | 0.780 | 0.857 | 0.841 | ... | 3.509 | 108.30 | 3.642 | 106.000 | 4.478 | 0.448 | 100.0 | 932.0 | 0.0 | 12.61 |
2016-01-09 19:00:00 | 9.950 | 9.880 | 10.050 | 9.970 | 9.910 | 9.870 | 0.561 | 0.469 | 0.639 | 0.547 | ... | 3.601 | 102.30 | 3.085 | 100.700 | 3.954 | 0.701 | 100.0 | 932.0 | 0.0 | 12.60 |
2016-01-09 19:10:00 | 9.620 | 9.600 | 9.590 | 9.550 | 9.240 | 9.260 | 0.767 | 0.666 | 0.847 | 0.732 | ... | 4.701 | 100.80 | 4.184 | 99.900 | 4.012 | 0.691 | 100.0 | 932.0 | 0.0 | 12.59 |
2016-01-09 19:20:00 | 9.590 | 9.600 | 9.510 | 9.460 | 9.270 | 9.270 | 0.615 | 0.571 | 0.795 | 0.760 | ... | 5.744 | 109.30 | 5.838 | 105.200 | 5.478 | 0.711 | 100.0 | 932.0 | 0.0 | 12.58 |
2016-01-09 19:30:00 | 10.520 | 10.520 | 10.520 | 10.440 | 10.510 | 10.490 | 0.608 | 0.571 | 0.501 | 0.477 | ... | 2.017 | 122.00 | 2.219 | 118.600 | 2.905 | 0.823 | 100.0 | 932.0 | 0.0 | 12.58 |
2016-01-09 19:40:00 | 8.970 | 8.950 | 9.190 | 9.100 | 9.260 | 9.190 | 0.691 | 0.617 | 0.705 | 0.659 | ... | 3.884 | 123.10 | 4.036 | 119.200 | 3.911 | 0.913 | 100.0 | 931.0 | 0.0 | 12.57 |
2016-01-09 19:50:00 | 7.334 | 7.339 | 7.212 | 7.151 | 6.995 | 6.964 | 1.286 | 1.283 | 1.470 | 1.423 | ... | 13.060 | 113.80 | 12.850 | 112.800 | 9.380 | 1.156 | 100.0 | 931.0 | 0.0 | 12.57 |
2016-01-09 20:00:00 | 5.310 | 5.445 | 4.788 | 4.901 | 4.093 | 4.190 | 1.202 | 1.190 | 1.259 | 1.226 | ... | 16.890 | 92.30 | 16.320 | 82.100 | 20.130 | 1.317 | 100.0 | 931.0 | 0.0 | 12.56 |
2016-01-09 20:10:00 | 4.052 | 4.044 | 3.956 | 3.921 | 3.572 | 3.500 | 0.881 | 0.868 | 0.802 | 0.787 | ... | 16.450 | 106.00 | 18.110 | 98.800 | 18.040 | 1.389 | 100.0 | 931.0 | 0.0 | 12.56 |
2016-01-09 20:20:00 | 3.632 | 3.519 | 3.653 | 3.584 | 3.594 | 3.524 | 0.816 | 0.787 | 0.658 | 0.615 | ... | 11.660 | 95.00 | 10.780 | 90.900 | 10.660 | 1.288 | 100.0 | 931.0 | 0.0 | 12.55 |
2016-01-09 20:30:00 | 6.868 | 6.797 | 6.667 | 6.566 | 6.363 | 6.277 | 1.297 | 1.313 | 1.383 | 1.371 | ... | 7.568 | 112.40 | 5.917 | 111.900 | 6.342 | 1.449 | 100.0 | 931.0 | 0.0 | 12.54 |
2016-01-09 20:40:00 | 3.496 | 3.462 | 4.098 | 4.110 | 4.252 | 4.318 | 1.363 | 1.367 | 1.616 | 1.593 | ... | 13.770 | 104.30 | 12.860 | 106.200 | 12.810 | 1.317 | 100.0 | 931.0 | 0.0 | 12.54 |
2016-01-09 20:50:00 | 2.390 | 2.394 | 2.033 | 2.000 | 1.402 | 1.347 | 0.774 | 0.706 | 0.725 | 0.682 | ... | 21.640 | 98.70 | 33.320 | 78.950 | 39.480 | 0.913 | 100.0 | 931.0 | 0.0 | 12.54 |
2016-01-09 21:00:00 | 3.002 | 3.042 | 2.509 | 2.521 | 2.252 | 2.156 | 1.313 | 1.265 | 1.237 | 1.285 | ... | 22.610 | 73.82 | 25.350 | 56.030 | 33.450 | 1.338 | 100.0 | 931.0 | 0.0 | 12.53 |
2016-01-09 21:10:00 | 3.489 | 3.448 | 3.061 | 3.031 | 2.874 | 2.851 | 0.821 | 0.793 | 0.632 | 0.575 | ... | 10.750 | 74.83 | 11.530 | 66.910 | 12.570 | 1.176 | 100.0 | 930.0 | 0.0 | 12.53 |
2016-01-09 21:20:00 | 4.204 | 4.321 | 3.661 | 3.715 | 2.972 | 3.074 | 0.995 | 0.924 | 1.049 | 1.005 | ... | 10.920 | 83.60 | 11.370 | 75.780 | 14.990 | 1.236 | 100.0 | 930.0 | 0.0 | 12.52 |
2016-01-09 21:30:00 | 6.486 | 6.648 | 5.745 | 5.879 | 4.862 | 5.021 | 1.224 | 1.237 | 1.329 | 1.329 | ... | 12.640 | 69.43 | 12.760 | 65.760 | 14.290 | 1.398 | 100.0 | 930.0 | 0.0 | 12.52 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2017-01-01 19:00:00 | 16.830 | 16.960 | 15.790 | 15.740 | 15.260 | 15.190 | 2.109 | 2.121 | 2.213 | 2.158 | ... | 5.864 | 275.20 | 0.000 | 24.220 | 7.789 | 0.246 | 100.0 | 975.0 | 0.0 | 13.07 |
2017-01-01 19:10:00 | 15.070 | 15.210 | 13.880 | 13.870 | 12.780 | 12.790 | 1.345 | 1.312 | 1.723 | 1.713 | ... | 4.644 | 275.20 | 0.000 | 27.490 | 5.928 | -0.097 | 100.0 | 975.0 | 0.0 | 13.07 |
2017-01-01 19:20:00 | 13.530 | 13.640 | 12.710 | 12.680 | 11.840 | 11.920 | 1.327 | 1.272 | 1.428 | 1.378 | ... | 4.862 | 275.20 | 0.000 | 27.340 | 5.738 | 0.003 | 100.0 | 976.0 | 0.0 | 13.07 |
2017-01-01 19:30:00 | 12.600 | 12.710 | 11.800 | 11.820 | 10.760 | 10.850 | 1.125 | 1.076 | 1.192 | 1.185 | ... | 3.664 | 275.20 | 0.000 | 26.710 | 5.446 | 0.124 | 100.0 | 976.0 | 0.0 | 13.06 |
2017-01-01 19:40:00 | 11.030 | 11.150 | 10.310 | 10.280 | 9.450 | 9.490 | 1.008 | 0.970 | 1.067 | 1.014 | ... | 4.025 | 275.20 | 0.000 | 27.130 | 6.349 | 0.225 | 100.0 | 976.0 | 0.0 | 13.06 |
2017-01-01 19:50:00 | 10.970 | 11.020 | 10.240 | 10.140 | 9.640 | 9.510 | 0.948 | 0.885 | 1.090 | 1.073 | ... | 4.460 | 275.20 | 0.000 | 24.730 | 6.135 | 0.398 | 100.0 | 976.0 | 0.0 | 13.06 |
2017-01-01 20:00:00 | 9.220 | 9.250 | 8.590 | 8.480 | 8.210 | 7.898 | 1.301 | 1.259 | 1.326 | 1.288 | ... | 6.344 | 275.20 | 0.000 | 16.530 | 8.410 | 0.408 | 100.0 | 976.0 | 0.0 | 13.05 |
2017-01-01 20:10:00 | 9.690 | 9.710 | 8.730 | 8.660 | 8.060 | 8.100 | 1.108 | 1.063 | 1.034 | 0.977 | ... | 8.260 | 275.20 | 0.000 | 17.780 | 10.360 | 0.427 | 100.0 | 976.0 | 0.1 | 13.05 |
2017-01-01 20:20:00 | 9.940 | 10.010 | 9.240 | 9.180 | 8.870 | 8.930 | 1.152 | 1.089 | 1.303 | 1.245 | ... | 6.100 | 275.20 | 0.000 | 14.710 | 8.140 | 0.458 | 100.0 | 976.0 | 0.0 | 13.05 |
2017-01-01 20:30:00 | 10.100 | 10.230 | 9.210 | 9.080 | 8.550 | 8.640 | 1.333 | 1.276 | 1.181 | 1.104 | ... | 6.533 | 275.20 | 0.000 | 16.060 | 9.860 | 0.418 | 99.5 | 977.0 | 0.0 | 13.04 |
2017-01-01 20:40:00 | 10.920 | 11.040 | 10.190 | 9.990 | 9.570 | 9.700 | 1.420 | 1.355 | 1.313 | 1.254 | ... | 5.977 | 275.20 | 0.000 | 21.060 | 7.317 | 0.418 | 99.0 | 977.0 | 0.0 | 13.04 |
2017-01-01 20:50:00 | 12.210 | 12.330 | 11.380 | 11.230 | 10.640 | 10.800 | 1.564 | 1.521 | 1.655 | 1.649 | ... | 4.017 | 275.20 | 0.000 | 25.220 | 5.931 | 0.317 | 98.2 | 977.0 | 0.0 | 13.04 |
2017-01-01 21:00:00 | 11.900 | 12.050 | 11.190 | 11.040 | 10.540 | 10.680 | 0.986 | 0.951 | 0.958 | 0.927 | ... | 4.436 | 275.20 | 0.000 | 22.000 | 6.066 | 0.306 | 98.5 | 977.0 | 0.0 | 13.04 |
2017-01-01 21:10:00 | 12.170 | 12.250 | 11.370 | 11.190 | 10.690 | 10.770 | 1.042 | 0.981 | 1.160 | 1.114 | ... | 4.543 | 275.20 | 0.000 | 20.370 | 5.186 | 0.427 | 98.3 | 977.0 | 0.0 | 13.03 |
2017-01-01 21:20:00 | 12.570 | 12.710 | 11.910 | 11.760 | 11.260 | 11.400 | 1.252 | 1.212 | 1.348 | 1.307 | ... | 3.612 | 275.20 | 0.000 | 23.020 | 5.229 | 0.317 | 96.9 | 977.0 | 0.0 | 13.03 |
2017-01-01 21:30:00 | 13.050 | 13.210 | 12.230 | 12.110 | 11.360 | 11.470 | 1.018 | 0.962 | 1.157 | 1.113 | ... | 3.574 | 275.20 | 0.000 | 23.330 | 5.919 | 0.367 | 97.1 | 977.0 | 0.0 | 13.03 |
2017-01-01 21:40:00 | 11.820 | 11.940 | 10.850 | 10.750 | 9.770 | 9.880 | 1.281 | 1.219 | 1.455 | 1.383 | ... | 4.477 | 275.20 | 0.000 | 21.990 | 6.635 | 0.225 | 96.0 | 978.0 | 0.0 | 13.02 |
2017-01-01 21:50:00 | 11.620 | 11.720 | 10.630 | 10.480 | 9.530 | 9.670 | 1.060 | 1.015 | 1.348 | 1.283 | ... | 4.358 | 275.20 | 0.000 | 19.960 | 6.322 | 0.043 | 95.7 | 978.0 | 0.0 | 13.02 |
2017-01-01 22:00:00 | 10.970 | 11.070 | 10.010 | 9.870 | 8.910 | 9.000 | 0.857 | 0.790 | 0.879 | 0.824 | ... | 4.366 | 275.20 | 0.000 | 21.390 | 6.312 | -0.087 | 95.8 | 978.0 | 0.0 | 13.02 |
2017-01-01 22:10:00 | 12.030 | 12.090 | 11.320 | 11.140 | 10.730 | 10.770 | 1.175 | 1.121 | 1.249 | 1.208 | ... | 3.851 | 275.20 | 0.000 | 21.520 | 5.347 | 0.033 | 95.9 | 978.0 | 0.0 | 13.01 |
2017-01-01 22:20:00 | 11.800 | 11.870 | 11.230 | 11.070 | 10.570 | 10.610 | 0.928 | 0.861 | 0.928 | 0.875 | ... | 3.375 | 275.20 | 0.000 | 21.520 | 5.000 | -0.168 | 93.9 | 978.0 | 0.0 | 13.01 |
2017-01-01 22:30:00 | 11.400 | 11.490 | 10.820 | 10.640 | 10.170 | 10.200 | 0.818 | 0.774 | 0.709 | 0.656 | ... | 3.046 | 275.20 | 0.000 | 22.250 | 4.233 | -0.159 | 94.2 | 978.0 | 0.0 | 13.01 |
2017-01-01 22:40:00 | 11.830 | 11.950 | 11.280 | 11.120 | 10.530 | 10.590 | 0.746 | 0.694 | 0.754 | 0.692 | ... | 3.629 | 275.20 | 0.000 | 23.010 | 4.384 | -0.178 | 93.1 | 978.0 | 0.0 | 13.01 |
2017-01-01 22:50:00 | 11.460 | 11.590 | 11.000 | 10.840 | 10.180 | 10.240 | 0.728 | 0.703 | 0.852 | 0.800 | ... | 2.977 | 275.20 | 0.000 | 19.010 | 5.281 | -0.219 | 93.5 | 978.0 | 0.0 | 13.00 |
2017-01-01 23:00:00 | 10.080 | 10.160 | 9.430 | 9.320 | 8.580 | 8.610 | 0.967 | 0.953 | 1.049 | 1.014 | ... | 4.395 | 275.20 | 0.000 | 12.240 | 9.010 | -0.391 | 93.8 | 979.0 | 0.0 | 13.00 |
2017-01-01 23:10:00 | 10.050 | 10.130 | 9.220 | 9.110 | 8.070 | 8.100 | 0.726 | 0.683 | 0.762 | 0.721 | ... | 3.568 | 275.20 | 0.000 | 15.050 | 7.453 | -0.512 | 95.5 | 979.0 | 0.0 | 13.00 |
2017-01-01 23:20:00 | 11.060 | 11.150 | 10.180 | 10.100 | 9.140 | 9.160 | 0.770 | 0.750 | 0.854 | 0.826 | ... | 2.853 | 275.20 | 0.000 | 12.760 | 6.748 | -0.624 | 96.1 | 979.0 | 0.0 | 13.00 |
2017-01-01 23:30:00 | 10.290 | 10.380 | 9.490 | 9.420 | 8.470 | 8.480 | 0.591 | 0.554 | 0.824 | 0.759 | ... | 3.826 | 275.20 | 0.000 | 11.380 | 7.505 | -0.705 | 96.8 | 979.0 | 0.0 | 13.00 |
2017-01-01 23:40:00 | 9.260 | 9.310 | 8.310 | 8.230 | 7.212 | 7.255 | 0.632 | 0.565 | 0.797 | 0.798 | ... | 6.536 | 275.20 | 0.000 | 9.160 | 8.400 | -0.877 | 96.8 | 979.0 | 0.0 | 12.99 |
2017-01-01 23:50:00 | 8.420 | 8.450 | 7.486 | 7.338 | 6.611 | 6.610 | 0.795 | 0.753 | 0.834 | 0.880 | ... | 5.014 | 275.20 | 0.000 | 7.084 | 14.140 | -0.887 | 97.1 | 979.0 | 0.0 | 12.99 |
48763 rows × 29 columns
These operations can also be performed on a specific column:
[10]:
data['Spd80mN']['2017-01-01':'2017-02-01']
[10]:
Timestamp
2017-01-01 00:00:00 5.876
2017-01-01 00:10:00 5.911
2017-01-01 00:20:00 7.004
2017-01-01 00:30:00 7.079
2017-01-01 00:40:00 6.796
2017-01-01 00:50:00 8.380
2017-01-01 01:00:00 8.910
2017-01-01 01:10:00 8.490
2017-01-01 01:20:00 7.116
2017-01-01 01:30:00 8.060
2017-01-01 01:40:00 9.150
2017-01-01 01:50:00 7.837
2017-01-01 02:00:00 6.234
2017-01-01 02:10:00 5.896
2017-01-01 02:20:00 4.897
2017-01-01 02:30:00 4.830
2017-01-01 02:40:00 4.728
2017-01-01 02:50:00 4.207
2017-01-01 03:00:00 4.315
2017-01-01 03:10:00 5.976
2017-01-01 03:20:00 6.539
2017-01-01 03:30:00 7.523
2017-01-01 03:40:00 7.904
2017-01-01 03:50:00 7.621
2017-01-01 04:00:00 7.791
2017-01-01 04:10:00 8.190
2017-01-01 04:20:00 8.200
2017-01-01 04:30:00 8.290
2017-01-01 04:40:00 8.230
2017-01-01 04:50:00 9.600
...
2017-02-01 19:00:00 9.980
2017-02-01 19:10:00 7.264
2017-02-01 19:20:00 8.210
2017-02-01 19:30:00 11.040
2017-02-01 19:40:00 12.240
2017-02-01 19:50:00 13.340
2017-02-01 20:00:00 14.150
2017-02-01 20:10:00 15.540
2017-02-01 20:20:00 14.870
2017-02-01 20:30:00 15.220
2017-02-01 20:40:00 14.510
2017-02-01 20:50:00 13.570
2017-02-01 21:00:00 13.200
2017-02-01 21:10:00 12.780
2017-02-01 21:20:00 13.230
2017-02-01 21:30:00 12.160
2017-02-01 21:40:00 11.400
2017-02-01 21:50:00 11.530
2017-02-01 22:00:00 11.940
2017-02-01 22:10:00 11.850
2017-02-01 22:20:00 10.240
2017-02-01 22:30:00 11.000
2017-02-01 22:40:00 11.900
2017-02-01 22:50:00 11.160
2017-02-01 23:00:00 10.580
2017-02-01 23:10:00 11.190
2017-02-01 23:20:00 11.580
2017-02-01 23:30:00 14.320
2017-02-01 23:40:00 12.870
2017-02-01 23:50:00 13.330
Name: Spd80mN, Length: 4608, dtype: float64
These ranges can then too be used within functions, such as monthly_means()
.
[11]:
bw.monthly_means(data['Spd80mN']['2017-01-01':])
[11]:
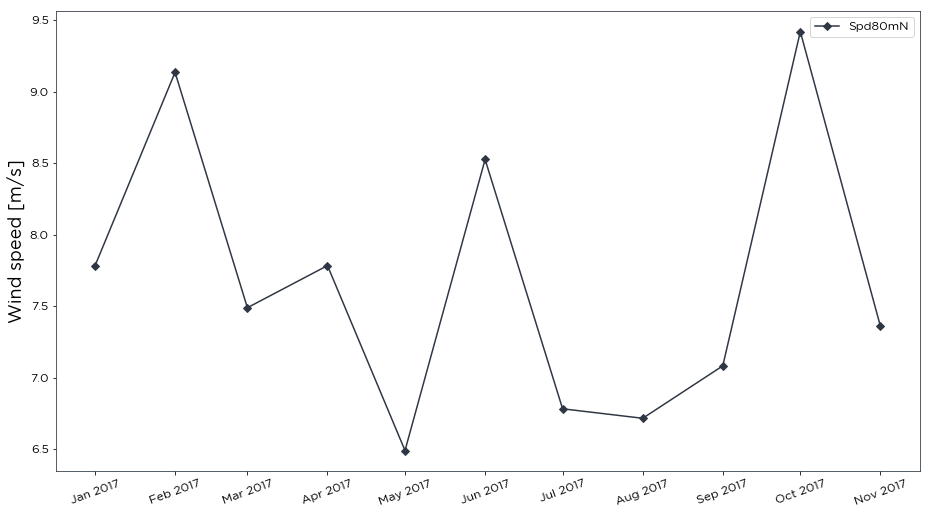
Step 3: Selecting Specific Entries¶
Specific entries in DataFrames and Series can be accessed both by their index and columns name, or by their position in the DataFrame or Series.
To select a specific entry by its columns name and index, i.e. the entry in the column Spd80mN at timestamp 2016-01-09 17:00:00, type:
[12]:
data['Spd80mN']['2016-01-09 17:00:00']
[12]:
7.652
To select a specific entry by its position in the DataFrame, i.e. the 3rd entry in the 1st column, use .iloc. When using Pandas, indexing starts at 0 for both columns and rows of DataFrames and Series. The index for the the 3rd entry in the 1st column would therefore be [2, 0]:
[13]:
data.iloc[2,0]
[13]:
7.652
There are many ways to slice and dice a pandas DataFrame making it a little confusing. These are just a few and are for most things all that are required.