How to export a tab file and save your data using the brightwind library¶
[1]:
import datetime
print('Last updated: {}'.format(datetime.date.today().strftime('%d %B, %Y')))
Last updated: 03 July, 2019
Outline:¶
This guide will demonstrate how to export data and a WAsP tab file using the following steps:
Import the brightwind library and some sample data
Plot a wind rose and produce a frequency table
Export the frequency table to a tab file for use in WASP or Openwind
Export the site data to a csv file
Import brightwind and data¶
[2]:
import brightwind as bw
[3]:
# specify location of existing sample dataset
filepath = r'C:\Users\Stephen\Documents\Analysis\demo_data.csv'
# load data as dataframe
data = bw.load_csv(filepath)
# apply cleaning
data = bw.apply_cleaning(data, r'C:\Users\Stephen\Documents\Analysis\demo_cleaning_file.csv')
# show first few rows of dataframe
data.head(5)
Cleaning applied. (Please remember to assign the cleaned returned DataFrame to a variable.)
[3]:
Spd80mN | Spd80mS | Spd60mN | Spd60mS | Spd40mN | Spd40mS | Spd80mNStd | Spd80mSStd | Spd60mNStd | Spd60mSStd | ... | Dir78mSStd | Dir58mS | Dir58mSStd | Dir38mS | Dir38mSStd | T2m | RH2m | P2m | PrcpTot | BattMin | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Timestamp | |||||||||||||||||||||
2016-01-09 15:30:00 | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | ... | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN |
2016-01-09 15:40:00 | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | ... | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN |
2016-01-09 17:00:00 | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | ... | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN |
2016-01-09 17:10:00 | 7.382 | 7.325 | 6.818 | 6.689 | 6.252 | 6.174 | 0.844 | 0.810 | 0.897 | 0.875 | ... | 4.680 | 118.8 | 5.107 | 115.6 | 5.189 | 0.954 | 100.0 | 934.0 | 0.0 | 12.71 |
2016-01-09 17:20:00 | 7.977 | 7.791 | 8.110 | 7.915 | 8.140 | 7.974 | 0.556 | 0.528 | 0.562 | 0.524 | ... | 3.123 | 115.9 | 2.960 | 113.6 | 3.540 | 0.863 | 100.0 | 934.0 | 0.0 | 12.69 |
5 rows × 29 columns
Plot a wind rose and produce a frequency distribution table¶
In order to export a tab file in the traditional format suitable for use in WAsP or openWind, it is first necessary to produce a frequency table using your desired anemometer and wind vane data. The brightwind library makes this simple. In the example below we pass the 80m anemometer and the 78m wind vane to the freq_table()
function. This automatically returns a nice wind rose plot of the site.
[4]:
bw.freq_table(data.Spd80mN, data.Dir78mS)
[4]:
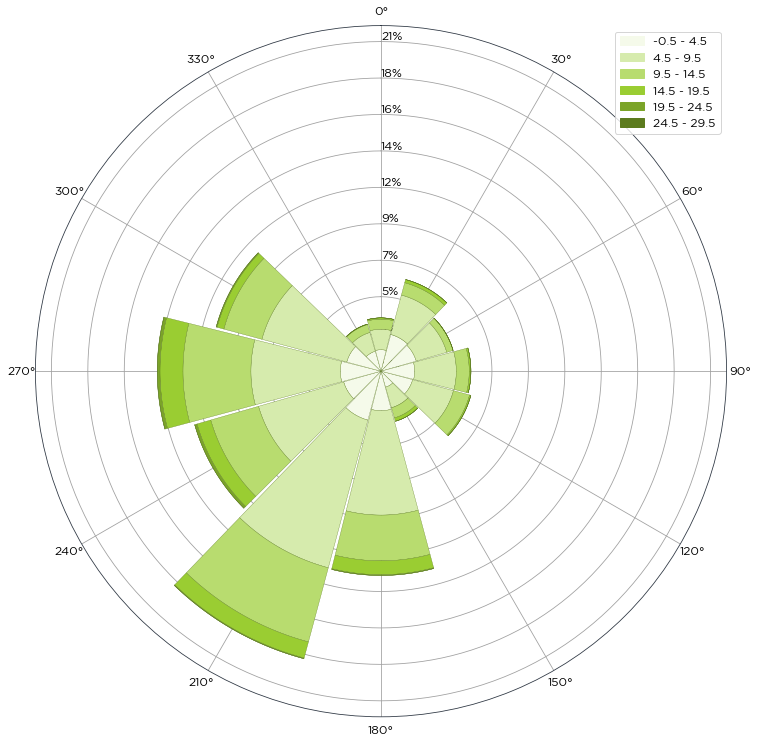
We want to return the data underpinning the windrose, i.e. the frequency table. To do this, we have to set the return_data variable to True in the function, and then set the function equal to a variable that represents the plot (Wind_rose) and the data (freq_table). The freq_table is then called for viewing.
[5]:
Wind_rose, freq_table = bw.freq_table(data.Spd80mN, data.Dir78mS, return_data=True)
[6]:
freq_table
[6]:
345.0-15.0 | 15.0-45.0 | 45.0-75.0 | 75.0-105.0 | 105.0-135.0 | 135.0-165.0 | 165.0-195.0 | 195.0-225.0 | 225.0-255.0 | 255.0-285.0 | 285.0-315.0 | 315.0-345.0 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
variable_bin | ||||||||||||
[-0.5, 0.5) | 0.049886 | 0.052380 | 0.115985 | 0.115985 | 0.150905 | 0.057369 | 0.135939 | 0.128456 | 0.150905 | 0.088547 | 0.047392 | 0.086053 |
[0.5, 1.5) | 0.214509 | 0.256912 | 0.276867 | 0.311787 | 0.329247 | 0.188319 | 0.244441 | 0.286844 | 0.270631 | 0.190814 | 0.217004 | 0.203285 |
[1.5, 2.5) | 0.324258 | 0.557475 | 0.614844 | 0.506342 | 0.497612 | 0.286844 | 0.500106 | 0.629809 | 0.495117 | 0.472669 | 0.432760 | 0.342965 |
[2.5, 3.5) | 0.370403 | 0.730828 | 0.689672 | 0.528790 | 0.501353 | 0.263148 | 0.589901 | 0.870509 | 0.724592 | 0.785703 | 0.647269 | 0.331741 |
[3.5, 4.5) | 0.384121 | 0.780714 | 0.596136 | 0.646022 | 0.572441 | 0.244441 | 0.998965 | 1.253383 | 0.788197 | 1.006448 | 0.871756 | 0.335483 |
[4.5, 5.5) | 0.337977 | 0.672212 | 0.562463 | 0.623574 | 0.543756 | 0.259407 | 1.374356 | 1.646234 | 0.986493 | 0.998965 | 1.012683 | 0.326753 |
[5.5, 6.5) | 0.349201 | 0.606114 | 0.492623 | 0.609855 | 0.563711 | 0.255665 | 1.552698 | 1.963010 | 1.222204 | 1.098737 | 1.158600 | 0.250677 |
[6.5, 7.5) | 0.301810 | 0.447726 | 0.381627 | 0.547498 | 0.532532 | 0.284350 | 1.401793 | 2.237382 | 1.283314 | 1.224699 | 1.146128 | 0.214509 |
[7.5, 8.5) | 0.184578 | 0.431513 | 0.321764 | 0.467680 | 0.587406 | 0.299315 | 1.199756 | 1.914371 | 1.162341 | 1.187284 | 1.104972 | 0.231969 |
[8.5, 9.5) | 0.109749 | 0.399087 | 0.233217 | 0.356684 | 0.420289 | 0.240699 | 1.008942 | 1.809611 | 0.891710 | 1.088759 | 1.078782 | 0.198296 |
[9.5, 10.5) | 0.107255 | 0.226981 | 0.195802 | 0.279361 | 0.346707 | 0.210768 | 0.814387 | 1.506554 | 0.791938 | 1.101231 | 0.798174 | 0.194555 |
[10.5, 11.5) | 0.152152 | 0.188319 | 0.094783 | 0.239452 | 0.283102 | 0.118479 | 0.582418 | 1.191026 | 0.783208 | 0.997718 | 0.563711 | 0.150905 |
[11.5, 12.5) | 0.170859 | 0.130950 | 0.076076 | 0.133445 | 0.192061 | 0.103513 | 0.541262 | 0.894205 | 0.627315 | 0.967786 | 0.405323 | 0.091042 |
[12.5, 13.5) | 0.114738 | 0.118479 | 0.026190 | 0.103513 | 0.130950 | 0.119726 | 0.485140 | 0.678448 | 0.541262 | 0.651011 | 0.360426 | 0.054874 |
[13.5, 14.5) | 0.087300 | 0.133445 | 0.013719 | 0.054874 | 0.087300 | 0.109749 | 0.438996 | 0.531285 | 0.366661 | 0.535026 | 0.335483 | 0.024943 |
[14.5, 15.5) | 0.056122 | 0.101019 | 0.003741 | 0.038662 | 0.039909 | 0.093536 | 0.275620 | 0.420289 | 0.321764 | 0.485140 | 0.183331 | 0.011224 |
[15.5, 16.5) | 0.013719 | 0.049886 | 0.001247 | 0.028684 | 0.026190 | 0.054874 | 0.233217 | 0.304304 | 0.244441 | 0.367908 | 0.125962 | 0.011224 |
[16.5, 17.5) | 0.007483 | 0.044897 | 0.001247 | 0.019954 | 0.016213 | 0.033673 | 0.198296 | 0.184578 | 0.165871 | 0.246935 | 0.069840 | 0.002494 |
[17.5, 18.5) | 0.001247 | 0.008730 | 0.000000 | 0.009977 | 0.002494 | 0.017460 | 0.107255 | 0.092289 | 0.104760 | 0.212015 | 0.034920 | 0.002494 |
[18.5, 19.5) | 0.001247 | 0.002494 | 0.000000 | 0.006236 | 0.000000 | 0.017460 | 0.053627 | 0.043650 | 0.056122 | 0.125962 | 0.027437 | 0.000000 |
[19.5, 20.5) | 0.000000 | 0.000000 | 0.000000 | 0.001247 | 0.000000 | 0.003741 | 0.023696 | 0.024943 | 0.041156 | 0.081065 | 0.016213 | 0.000000 |
[20.5, 21.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.011224 | 0.012471 | 0.037414 | 0.033673 | 0.022449 | 0.000000 |
[21.5, 22.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.007483 | 0.004989 | 0.038662 | 0.031179 | 0.004989 | 0.000000 |
[22.5, 23.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.003741 | 0.001247 | 0.024943 | 0.009977 | 0.007483 | 0.000000 |
[23.5, 24.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.001247 | 0.000000 | 0.008730 | 0.007483 | 0.002494 | 0.000000 |
[24.5, 25.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.006236 | 0.004989 | 0.000000 |
[25.5, 26.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.002494 | 0.002494 | 0.001247 | 0.000000 |
[26.5, 27.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.002494 | 0.002494 | 0.000000 | 0.000000 |
[27.5, 28.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.001247 | 0.000000 | 0.000000 | 0.000000 |
[28.5, 29.5) | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.001247 | 0.000000 | 0.000000 |
Export a tab file¶
Once a frequency table has been generated, producing a tab file is just one line of code. The export_tab_file()
function requires the frequency table (freq_table) as an input, along with the height above ground level of the distribution (80m), and the latitude (56) and longitude (-4) coordinates of the site. The filename is specified by setting the file_name variable. The tab file will be outputted in the folder containing the jupyter notebook. The folder_path variable can be set if the user
would like to export the tab file to another folder.
[7]:
bw.export_tab_file(freq_table, 80, 56, -4, file_name='Irish_Site_1')
Export of tab file successful.
If you want to export the file to a particular location other than the location of your working Jupyter Notebook you can add in the file path.
[8]:
export_folder = r'C:\Users\Stephen\Documents\Analysis'
bw.export_tab_file(freq_table, 80, 56, -4, file_name='Irish_Site_1', folder_path=export_folder)
Export of tab file successful.
Export the site data to a csv file¶
To export the data to a location of your choice, simply pass the dataframe representing the site data into the export_csv()
function and set the file_name variable to your name of choice. This will output the data into a file in the same folder as your jupyter notebook. The folder_path variable can be set if the user would like to export the data to another folder.
[9]:
bw.export_csv(data, file_name='Demo_data.csv')
Export to csv successful.